Engineering View Architecture and APIs
Architecture
The following diagram shows interactions between components in the Engineering View. The blue numbers are bugs describing the tasks to be implemented.
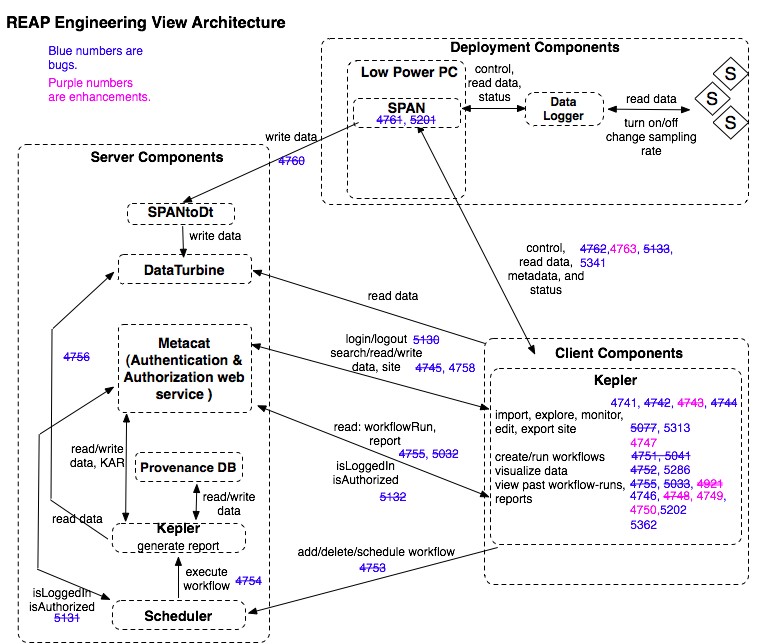
APIs
An API to control sensors.
// Turn on or off a sensor void setSensorOn(String sensorName, String dataLoggerName, boolean isOn) throws Exception // Set the sampling period for a sensor void setSensorSampling(String sensorName, String dataLoggerName, int frequency) throws Exception
An API to query and receive changes about metadata.
// Get a list of sensors List<String> getSensorNames() throws Exception // Get a list of names of data loggers. List<String> getDataLoggerNames() throws Exception // Get the name-value metadata for a sensor Map<String,String> getMetadataForSensor(String sensorName, String dataLoggerName) throws Exception // Set a metadata name-value for a sensor. void setMetadataForSensor(String sensorName, String dataLoggerName, String name, String value) throws Exception // Returns true if sensor is on. boolean getSensorOn(String sensorName, String dataLoggerName) throws Exception // Get the sampling period for a sensor int getSensorSampling(String sensorName, String dataLoggerName) throws Exception // Start receiving metadata changes from all sensors into a queue. // Once this method is called, metadata changes are continually added // to the queue until stopReceivingMetadata() is called with // the same queue. void startReceivingMetadata(Queue<SensorMetadata> queue) // Stop receiving sensor metadata changes into a queue. void stopReceivingMetadata(Queue<SensorMetadata> queue)
An API to receive data.
// Start receiving data values from all sensors into a queue. // Once this method is called, data are continually added // to the queue until stopReceivingData() is called with // the same queue. void startReceivingData(Queue<SensorData> queue) // Stop receiving sensor data into a queue. void stopReceivingData(Queue<SensorData> queue) // Get the last value from a specific sensor String getLastValue(String sensorName, String dataloggerName)
An API for the connection. Since the sensor server may limit the number of connections, methods to maintain a reference count are provided so that a single connection may be shared.
// Increment the reference count void incrementRefCount() // Decrement the reference count void decrementRefCount() throws Exception // Disconnect void disconnect() throws Exception // Returns true if connected boolean isConnected()